Email validation is one of the most common tasks in web development. In this tutorial, I will show you at least 3 ways to validate an email in Python.
This tutorial covers 3 ways to validate an email in Python:
- Using RegEx.
- Using the
email_validator
library (recommended). - Using the
flanker
library.
Let's find out how to use those methods to validate an email in Python and compare their performance at the end of this tutorial.
Contents
- Check if an Email is Valid using RegEx
- Check if an Email is Valid using the
email_validator
Library - Check if an Email is Valid using the
flanker
Library - Comparison
- Conclusion
Check if an Email is Valid using RegEx
A RegEx pattern is a sequence of characters that define a search pattern. It's used to match character combinations in strings.
Similar to extracting URLs in a Python string, we can also produce a RegEx pattern to validate an email.
Take a look at those example email addresses:
dminhvu.work@gmail.com
dminhvu+abc@gmail.com
minhvdse000@fpt.edu.vn
The common pattern among those email addresses is:
- Starts with some letters, numbers, or special characters like
.
or+
, e.g.dminhvu
,dminhvu+abc
,minhvdse000
. - Followed by an
@
symbol. - Followed by a domain name, e.g.
gmail.com
,fpt.edu.vn
.
Based on common email patterns, we can create a RegEx pattern to validate an email: ^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$
.
Let's break it down:
- The first and the last characters:
^
and$
are the start and end of the string, respectively. - The first part:
[a-zA-Z0-9_.+-]+
matches one or more letters, numbers, or special characters like.
or+
. - The second part:
@
matches the @ symbol. - The third part:
[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+
matches a domain name.
Here is the Python code that applies the above RegEx pattern to validate an email:
import re def validate_email(email): pattern = r"^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$" return re.match(pattern, email) print(validate_email("dminhvu")) # None print(validate_email("dminhvu.work@gmail.com")) # <re.Match object; span=(0, 24), match='dminhvu.work@gmail.com'> print(validate_email("dminhvu+abc@gmail.com")) # <re.Match object; span=(0, 21), match='dminhvu+abc@gmail.com'> print(validate_email("minhvdse000@fpt.edu.vn")) # <re.Match object; span=(0, 24), match='minhvdse000@fpt.edu.vn'>
You can run it using the python3 main.py
command.
The re.match
function returns a match object if the email is valid. Otherwise, it returns None
.
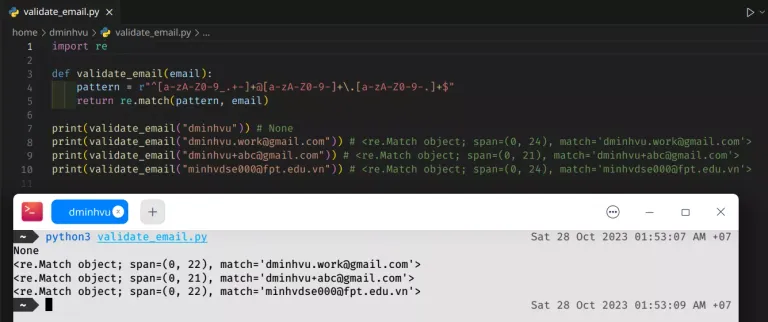
Check if an Email is Valid using the email_validator
Library
The email_validator
library is a simple library to validate email addresses. You can use it to check if an email is valid or not. This is by far the library I mostly use to validate emails in Python.
To use the email_validator
library, follow these steps:
-
Go to Terminal or Command Prompt.
-
Run the following command:
Terminal pip install email-validator
-
Create a Python file, e.g.
main.py
. -
Use the following code to validate an email:
main.py from email_validator import validate_email, EmailNotValidError def validate_email_address(email): try: # Validate. emailinfo = validate_email(email, check_deliverability=True) # Replace with the normalized form. email = emailinfo.normalized return email except EmailNotValidError as e: # email is not valid, exception message is human-readable print(str(e)) return None print(validate_email_address("dminhvu")) # The email address is not valid. It must have exactly one @-sign. print(validate_email_address("dminhvu.work@gmail.com")) # dminhvu.work@gmail.com
You can run it using the python3 main.py
command.
Check if an Email is Valid using the flanker
Library
The flanker
library is a powerful library to validate email addresses developed by the Mailgun Team. It can also parse email addresses, normalize them, and extract the domain part.
To use the flanker
library, follow these steps:
-
Go to Terminal or Command Prompt.
-
Run the following command to install the
flanker
library as its dependencies (e.g.redis
,dnsq
):Terminal pip install flanker redis dnsq
-
Create a Python file, e.g.
main.py
. -
Use the following code to parse an email:
main.py from flanker.addresslib import address def parse_email_address(email): return address.parse(email) print(parse_email_address("dminhvu")) # None print(parse_email_address("dminhvu.work@gmail.com")) # dminhvu.work@gmail.com
If you see it returns an email, it means the email is valid. Otherwise, it returns None
.
To validate an email, you will need to have a Redis server running first. Then you can use the address.validate_address
function to validate an email. However, I haven't tried this method yet, so please let me know if it works for you.
Comparison
Here is a comparison of the 3 methods:
Method | Pros | Cons |
---|---|---|
RegEx |
|
|
email_validator |
|
|
flanker |
|
|
In general, I recommend using the email_validator
library to validate an email in Python. It's easy to use and has a fast performance.
Conclusion
In this tutorial, we have learned at least 3 ways to validate an email in Python. We used RegEx and 2 libraries: email_validator
and flanker
.
I hope you find this tutorial helpful. If you have any questions, feel free to leave a comment below.
Comments
Be the first to comment!